Objectives:
- Build PHP pages
- Apply loops (For, While, & Do-While)
- Apply if, else if, and switch statements
- Apply functions
- Learn about integrating HTML, PHP, and CSS together
- Dynamic coding
Point Value:
Specifics:
- Chapters:
- 8: starting page 234
- 13:starting page 382
- Downloads:None
- References:
- SSP03 Date Sample (http://apollo.occc.edu/chyde/cs2623/assignments/ssp03_date_example.txt): Note, change the ".txt" file extension to ".php" if you want to run and test.
- Collaboration Assignment: On the forumn, explain which you like more. The switch or the if else/if statement. Do the same for the loops (i.e. which of the three loops do you like the most). For the loops which are testing their conditions pre and post. At the very least: which do you like, why, and what do you not like about the others. other.
-
SSP03:
- Notes:
-
Use the following code to get the current day:
"$current_day = date('N');" -
For the purpose of this assignment, the numbers associated with the day of the week will be.
Days of Week Num String 1 Monday 2 Tuesday 3 Wednesday 4 Thursday 5 Friday 6 Saturday 7 Sunday - The school apollo1 server, and likely yourr local server, have the timezone set to UCT which is +6 hours (USA Central Time zone). I do not want you losing time resetting this so just be aware of this setting when doing your assignment.
-
Use the following code to get the current day:
-
Functions
- Folder: create a folder entitled functions in your root PHP directory.
- File: create a file called functions_ssp03.php in that folder.
- fn_tableOpen(): Create a function entitled fn_tableOpen(). This function will have one paramater for the tables caption. It will contain static code to open a table, apply your CSS formatting class(es), and create the header columns. Your header columns will be the same as what you did in SSP02 which were Day, Details, and Time. Note, when populating the day column with the functions below you will use the string (e.g. "Monday") instead of the number (e.g. "1").
- fn_tableClose(): Create a function entitled fn_tableClose(). THis function will not have any paramaters. It will contain static code for a table closing tag followed by two line breaks (e.g. <br>).
-
fn_tableRowCurrent(): Create a function entitled fn_tableRowCurrent(). This function
will have three paramaters for the three different columns. You will have the approrpriate code to display these paramaters
in a new row.
Also, it will have css coding to highlight the row. By that I mean change this rows background color to yellow (e.g. #FFFF66). Sorry in advance if that does not align with your pages color schema. I need something standard for grading purposes. - fn_tableRow(): Create a function entitled fn_tableRow(). This will be the same as fn_tableRowCurrent() except you will NOT have the special highlighting.
-
fn_dayOfWeek(): Create a function entitled fn_dayOfWeek(). This will have one
parameter for the numerical day of the week. You will return the actual string day of the week. For example, if
you get a 1 it will be "Monday", 2 it will be "Tuesday", etc. Reference the table above!
I expect you to use an if staement with a else if for this. - fn_dayDetails(): Create a function entitled fn_dayDetails(). Use a switch statement, create details about your CS2623 study goals for eadh day of the week. The function will take one parameter which is the numerical day of the week. For this assignment, you must dedicate at least four days to the course with a certain amount of study time for each day.
- fn_dayTime(): Create a function entitled fn_dayTime(). Using an if else/if statement, write code to give the amount of time you will study on CS2623 for days of the week. For two of your four days, the study time will be the same. The other two must be unique. For the ones with the same day, you must use an "OR" the logical operator.
- Loops:
- Folder: Create folder in your root directy entitled SSP03.
- File: Create a copy of your SSP02.php file and name it SSP03. Save it in the SSP03 folder.
- Navigation: Update your navigation to include the SSP03 page on your SSP03.php.
- Content: Remove the include dymanic content from your body. Where it was in your SSP03.php file you will be entering PHP information for the following loops.
- For Loop: Create a table with a for loop for each day of the week. Your table should start on Monday and go to Sunday. Your caption should be "CS2623: <YourName> Weekly Planner" where <YourName> will be your actual name. The tables content and syntax should be done with the functions (minus the loop syntax). If the rows day is the current day than use fn_tableRowCurrent() for the day rows. Otherwise, you would use fn_tableRow().
- While Loop: Create a table with a while loop for the weekdays only. A weekday is Monday to Friday. Your caption should be "CS2623: <YourName> Weekday Planner" where <YourName> will be your actual name. The tables content and syntax should be done with the functions (minus the loop syntax). If the rows day is the current day than use fn_tableRowCurrent() for the day rows. Otherwise, you would use fn_tableRow().
-
Do-While Loop: Create a table with a do-while loop that starts from today and ends
on Sunday. Your caption should be
"CS2623: <YourName> To-Do Planner" where <YourName> will be your actual name.
Note, this table will end up being dynamic in that the number of rows will differ from day to day. If it's Sunday you should still see a row for Sunday.
- Upload Code: Upload your files/folders to Apollo1 and test your code. Submit a link to your SSP03.php page via moodle. Make sure to look at your source code. The HTML code generated by your functions should have appropriate line breaks, indention, etc. Finally, your functions and code should have appropriate comments
- Notes:
Helpful Illustrations
Sample function
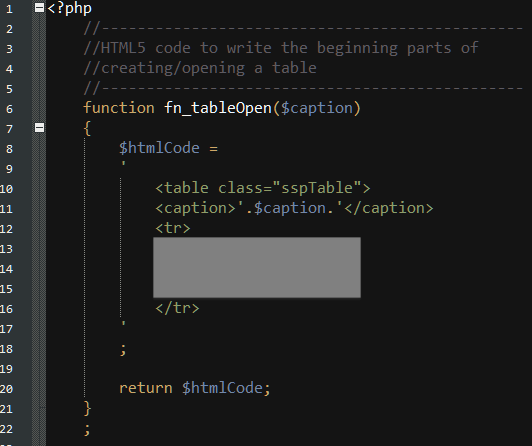
Sample table Output: Browser View
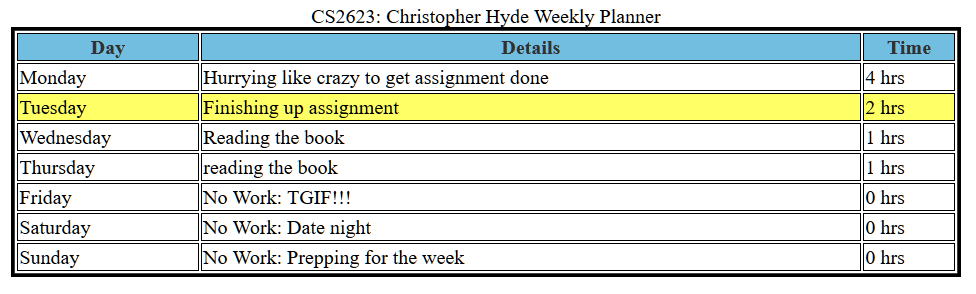
Sample table Output: Browser Source View
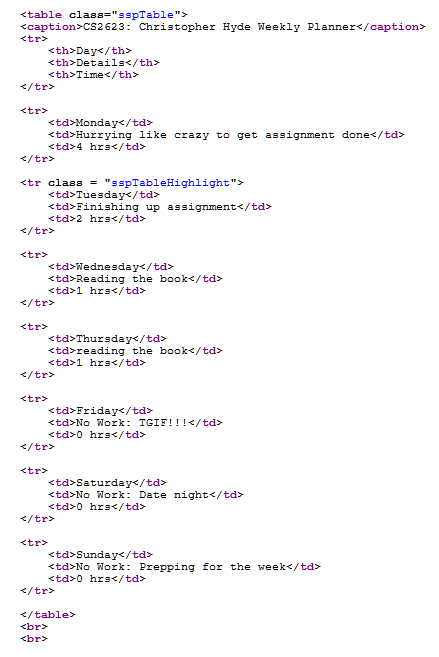
Sample table Output: PHP File
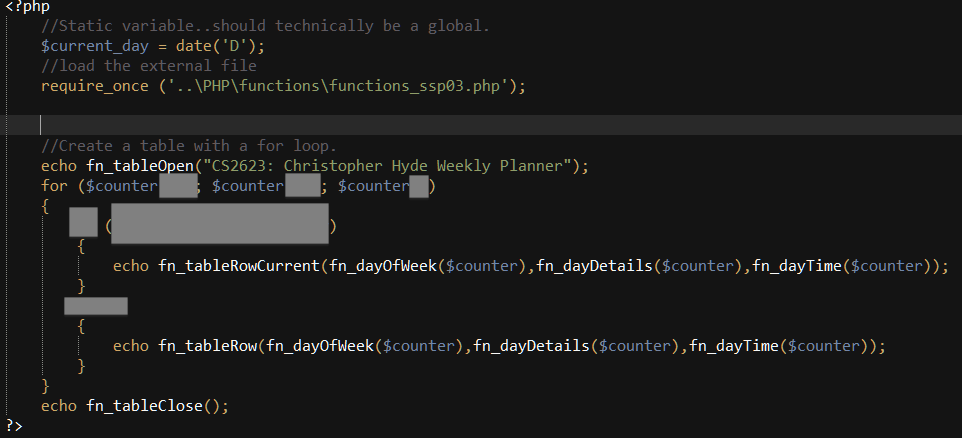
Common Requirements for All Web Pages:
- Your code must adhere to HTML5 standards. W3C HTML5 Example: https://www.w3schools.com/html/html5_intro.asp
- All your pages must pass W3C validators
- HTML Validator: https://validator.w3.org/nu/
- CSS Validator: http://jigsaw.w3.org/css-validator/
- The web pages must display correctly in all of the following: FireFox, Chrome, Internet Explorer.
- The links between the existing pages with the Pacific Trails Resort website cannot be broken.
-
All HTML and CSS code must be well documented, properly indented, and easy to read.
http://css-tricks.com/examples/CleanCode/Beautiful-HTML.png
http://coding.smashingmagazine.com/2008/05/02/improving-code-readability-with-css-styleguides/